My learning journey of JavaScript fundamentals
What is JavaScript and what is its relationship to HTML and CSS?
In web development, HTML defines the structure of your content, CSS determines the style and layout, and JavaScript makes the content interactive. None of them are dispensable.
Let's consider that building a good-looking and fully functional website is like building your dream car. The first step is to build the frame of the car, and your friend, Mr. HTML, helps you with it. Then, you want your dream car to be unique! Your other friend, Ms. CSS, adds color with hex digits to the car's frame, designs special big fancy rims, new-tech LED headlights, and more. Cool! Your car looks awesome!
You decide to show off your car, but wait, there's no engine! No brakes! No transmission! All of the functional parts are missing. Right now, it's just a good-looking car frame! Luckily, Mr. HTML and Ms. CSS introduce their friend, Mr. JavaScript, who comes over to help you add all the needed functional parts to your car. Phew! The car is finally done, and it's time to show off!

Control flow and Loops
Control flow is how the computer runs the code from top to bottom in JavaScript. It nomally starts from the first line and ends at the last line. However, some statement can changes the control flow, such as conditionals, loops and functions.
There is a interesting programmer joke:
Wife: After you're done with work, make sure to buy a dozen of eggs. And if they have bread, go ahead and buy one.
Husband: Alright.
Later that night...
Wife: Why did you only buy one egg?
Husband: Well, I spotted they having bread, so...
Lol! You may not understand why this husband does not follow his wife's
instructions. First, let's find out what the conditional statement is.
Conditional statements is used for determining whether or not pieces of
code can run. In JavaScript, we have if
,
else if
, else
and
switch
statements.
if
statement
Let's putting the wife's instructions with if
statement,
you can see that:
let buyEggs = 12; let haveBread = true; if(haveBread){ buyEggs = 1; }
Wow! After this if
statement, the buyEggs
will
return to 1 instead of 12. Because the condition
haveBread
is true and is used to specify execution for a
block of code buyEggs = 1;
. Now you may understand why the
husband will only buy one egg. He must be a programmer!
if else
statement
Let's consider other conditional statements with the wife's different instructions for her programmer husband.
Wife: go to the store and buy a dozen of eggs. If they have bread, buy one. If they do not have bread, buy extra a dozen of eggs.
Following wife's instructions, here is the code:
let buyEggs = 12; let haveBread = true; if(haveBread){ buyEggs = 1; }else{ buyEggs += 12; }
The husband will buy either 1 or 24 eggs based on whether the store has bread.
else if
statement and switch
statement
The else if
statement and the switch
statement
are similar in some ways, but they have distinct differences. The key
distinction is that the else if
statement allows for
specifying a new condition to test if the previous condition evaluates
to false. On the other hand, the switch
statement is used
to determine the matching expression among several possibilities.
let's check wife's new instruction: go to the store and buy a dozen of eggs. If they have bread, buy one. If they have apples, buy five. If they do not have any, buy extra a dozen of eggs.
Here is the code with else if
statement:
let buyEggs = 12; let haveBread = true; let haveApple = ture; if(haveBread){ buyEggs = 1; }else if(haveApple){ buyEggs = 5; }else{ buyEggs += 12; }
Here is the code with switch
statement:
let buyEggs = 12; let haveBread = true; let haveApple = ture; switch(condision){ case haveBread: buyEggs = 1; break; case haveApple: buyEggs = 5; break; default: buyEggs += 12; }
The husband will buy either 1, 5 or 24 eggs based on what he seeing in the store. What a great husband!
Loops
Loops are one of the important control flows in JavaScript and can execute a block of cose a number of a times. It is similar to repeating a simple task for many times in the life. For example, you decide to serve 20 beautiful sunny side up eggs for your guests and you only have one pan, so you have to repeat the procedures: cracking the egg, pan-frying it, and serving it until you feed all the guests.
If we put this example into JavaScript, the code will be liked:
for(let i = 0; i < 20; i++){ text += egg[i] + " is done!" + <br>; }
The code above is using the for
loops. There are threee
expressions in side the parentheses:
-
Expression 1:
let i = 0;
is executed only one time before the execution of the code block. It likes a note to remind that you should start to cook the egg. But be careful, the computer program counts index starting from 0. -
Expression 2:
i < 20;
definds the condition for executing the code block. It is an another note to remind you how many eggs you should cook. -
Expression 3:
i++
is executed every time after the code block has been executed. It is a counter to count how many eggs have been cooked.
We have anothe way while
to execute the loops.
let i = 0; while (i < 20){ text += egg[i] + " is done!" + <br>; i++; }
The code in while
loops will run over and over again until
the variable i
equals to 20. The i
is a
counter to remind you how many eggs have been cooked!
What is the DOM? How do we interact with it?
The DOM is from abbreviation of Document Object Model, a powerful tool and a programming interface for web documents. The programmers can use it to represent the web page so that programs can change the document structure, style and content.
The HTML DOM is constructed as a tree of Objects:
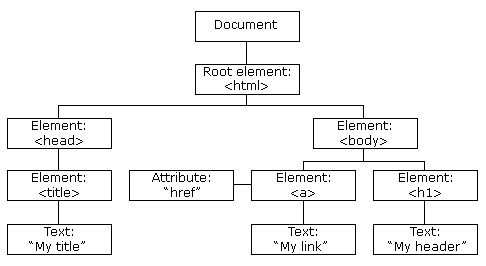
The reason why we should use DOM representation is because it allows us to manipulate the web page, and it can be modified with a scriping language such as JavaScript. For example, we can change the content of an element with an id:
<html> <body> <p id = "example"></p> <script> document.getElementById("examaple").innerHTML = "Hello!"; </script> </body> </html>
Also, we can use many the DOM specified methods, such as
querySelector
, getElementById
,
addEventListener()
and createElement()
etc.
The DOM is our useful tool in our learning journey!
The difference between accessing data from arrays and objects.
Objects
What are objects in JavaScript? They are mutable data structure in JavaScript to represent a thing, and it can be anything: a car, a person, a bird etc. We can store the data in key value pair. The key is itetable and can be anything. Here is the code how to create an object:
const person = { name: 'Yuekai Wu' age: '27' gender: 'male' }
The way to access the value of the object is easy, we can either a dot
operator followed by key name person.name
or a square
bracket along with key in string format person['name']
.
To modify the value to an object:
person.age = 28; //returns 28
To add a value to an object:
person.hobbies = 'Playing video game';
To delete a value from an object:
delete person.age;
Arrays
Arrays are a kind of objects in JavaScript. They store the data in an ordered collection in which the data can be accessed using a numerical index. It is the major difference to the objects. They are also mutable and data can be modified at any index. However, we need to be careful that the indexes of arrays are zero based, meaning that the first item is stored in zero index, the second item at first index and so on. It is so different from our brain! Here is the code how to create an array:
//index [0][1][2][3][4][5] const number = [1, 2, 3, 4, 5, 6];
To modufy the value of index '1' to an array:
number[1] = 10; //returns 10
To add a value to the front an array:
number.unshift(0);
To add a value to the end an array:
number.push(7);
To remove the value from an array:
delete number[3]; //returns [1, 2, 3, undefined, 5, 6]
Be careful that removing the element form the array by
delete
, this method will replace the value with undefined
instead of delete the index.
Conclusion
If you want to store the data in oder or in a sequence, use arrays. Otherwise, just uss objects for everything else.
What are functions? Why are they helpful?
A JavaScript function is a block of code designed to perform a particular task. To achieve this part, we need to declare a funtion:
function myAddingFunction(num1, num2){ return num1 + num2; }
The code above is defined with the function
keyword and
followed by a name myAddingFunction
. Also, the followed
parentheses include the parameters num1
and
num2
. The code return num1 + num2;
to be
executed is place inside the curly brackets by the function.
You may wonder that why the function has been declared, but there is nothing on the screen. It is simple, the function is only executed when you call it! How to call it? Let's check the code:
let number = myAddingFunction(65, 32); //return 97 number = myAddingFunction(25, 32); //return 57
You can see that I just change the value of a parameter, and the element
number
will return to different value after it calls the
function. The code in function will perform the adding task for
number
.
Let's consider your washing machine. With it, you don't have to do much. Just put your clothes in and press the start button. The washing machine will take care of washing the clothes for you. Functions, once declared, can be called at any time and from anywhere you need them. They serve as a valuable tool that can save both your work and your life!
30 JUNE 2023